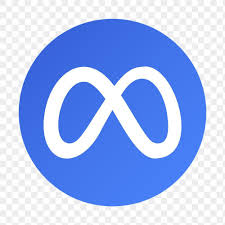
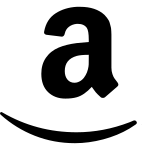
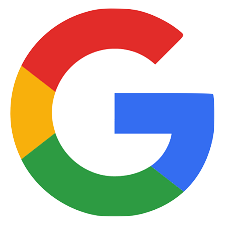
(This question has been seen in the interviews of the following companies: Facebook, Amazon, Google)
Give a binary tree, find if it's possible to cut the tree into two halves of equal sum. You can only cut one edge.
Solution:
public boolean cutTree(TreeNode root) {
if(root == null) {
return false;
}
List sumArr = new ArrayList<>();
int sum = subTreeSum(root, sumArr);
if ((sum & 1) == 0) {
for (int i = 0; i < sumArr.size() - 1; i++) {
if (sumArr.get(i) == (sum >> 1)) {
return true;
}
}
}
return false;
}
public int subTreeSum(TreeNode node, List sumArr) {
int result = node.val;
if(node.left != null) {
result += subTreeSum(node.left);
}
if(node.right != null) {
result += subTreeSum(node.right);
}
sumArr.add(result);
return result;
}
Get one-to-one training from Google Facebook engineers
Top-notch Professionals
Learn from Facebook and Google senior engineers interviewed 100+ candidates.
Most recent interview questions and system design topics gathered from aonecode alumnus.
One-to-one online classes. Get feedbacks from real interviewers.
Customized Private Class
Already a coding expert? - Advance straight to hard interview topics of your interest.
New to the ground? - Develop basic coding skills with your own designated mentor.
Days before interview? - Focus on most important problems in target company question bank.