The Fulfillment Center consists of a packaging bay where orders are automatically packaged in groups(n). The first group can only have 1 item and all the subsequent groups can have one item more than the previous group. Given a list of items on groups, perform certain operations in order to satisfy the constraints required by packaging automation.
The conditions are as follows:
-The first group must contain 1 item only.
-For all other groups, the difference between the number of items in adjacent groups must not be greater than 1. In other words, for 1<=i<n, arr[i]-arr[i-1]<=1
To accomplish this, the following operations are available:
- Rearrange the groups in any way.
- Reduce any group to any number that is at least 1
Write an algorithm to find the maximum number of items that can be packaged in the last group with the conditions in place.
Input
The function/method consists of two arguments:
numGroups, an integer representing the number of groups(n);
arr, a list of integers representing the number of items in each group
Output
Return an integer representing the maximum items that can be packaged for the final group of the list given the conditions above.
Example1:
Input:
[3,1,3,4]
Output:
4
Explanation:
Subtract 1 from the first group making the list [2, 1, 3, 4]. Rearrange the list into [1, 2, 3, 4]. The final maximum of items that can be packaged in the last group is 4.
Example2:
Input:
[1,3,2,2]
Output:
3
Example3:
Input:
[1,1,1,1]
Output:
1
Example4:
Input:
[3,2,3,5]
Output:
4
Solve the problem:
Python3Solution
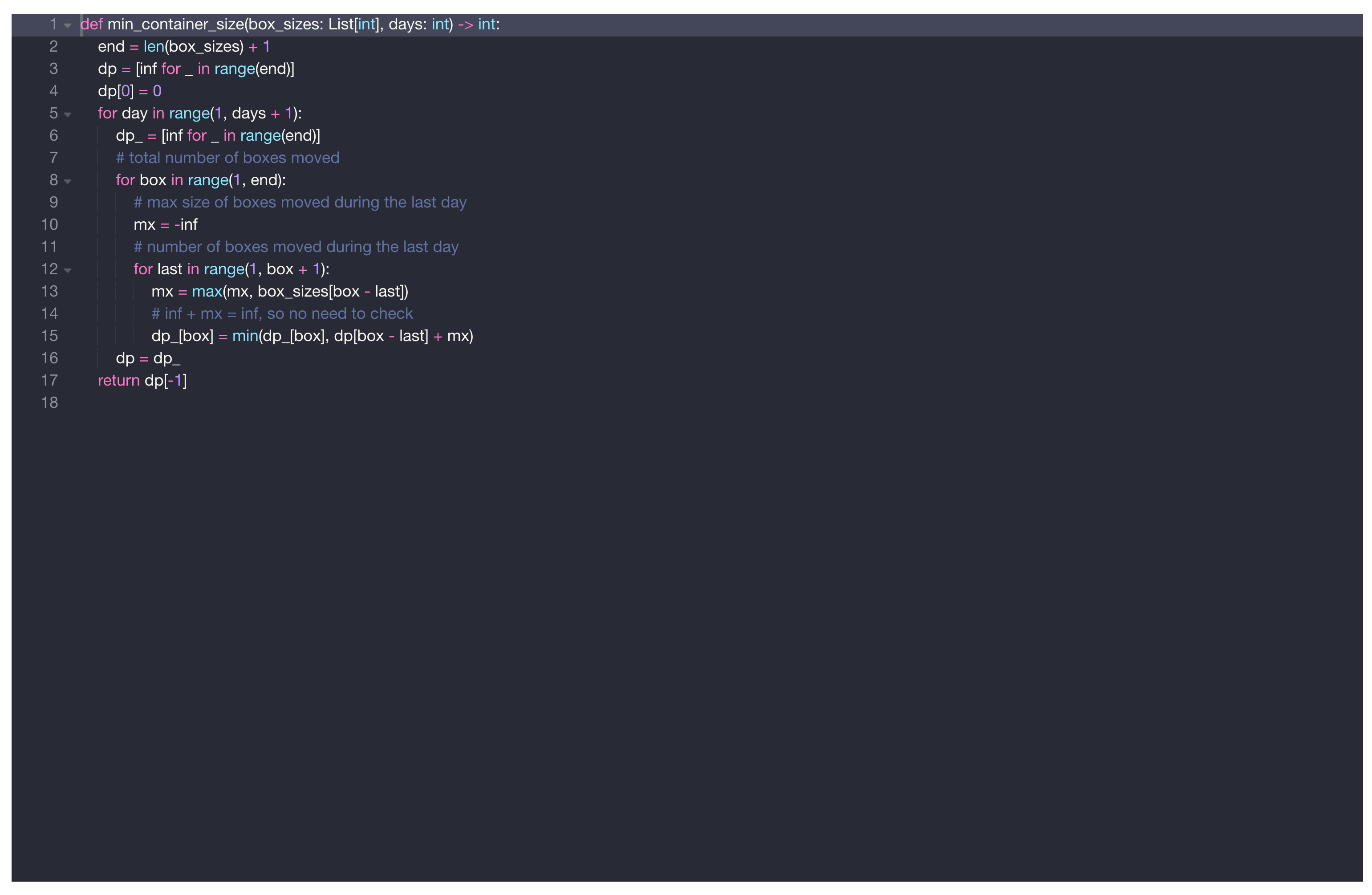
Get one-to-one training from Google Facebook engineers
Top-notch Professionals
Learn from Facebook and Google senior engineers interviewed 100+ candidates.
Most recent interview questions and system design topics gathered from aonecode alumnus.
One-to-one online classes. Get feedbacks from real interviewers.
Customized Private Class
Already a coding expert? - Advance straight to hard interview topics of your interest.
New to the ground? - Develop basic coding skills with your own designated mentor.
Days before interview? - Focus on most important problems in target company question bank.